Object-Oriented Programming (OOP) in PHP: Building User-Centric Applications
Building Blocks of OOP: Classes and Objects in PHP
Object-Oriented Programming (OOP) is a powerful programming paradigm that revolutionizes how we structure and organize code. PHP, a versatile server-side scripting language, fully embraces OOP principles, enabling developers to create modular, reusable, and maintainable code that translates to enhanced user experiences.

Understanding Classes and Objects
- Classes: Classes serve as blueprints or templates for creating objects. They define the properties (data) and methods (functions) that an object will possess.
- Objects: Objects are instances of classes. They represent real-world entities or abstract concepts within your application.
Let’s look at a basic example:
class User {
public $name;
public $email;
public function __construct($name, $email) {
$this->name = $name;
$this->email = $email;
}
public function greet() {
echo "Hello, " . $this->name . "!";
}
}
$user1 = new User("Alice", "[email protected]");
$user1->greet(); // Output: Hello, Alice!
Key OOP Concepts
- Encapsulation: Bundling data (properties) and the methods that operate on that data within a single unit (the class). This promotes data integrity and security.
- Inheritance: Creating new classes (child classes or subclasses) that inherit properties and methods from existing classes (parent classes or superclasses). This fosters code reusability and establishes hierarchical relationships. PHP
class AdminUser extends User { public function accessAdminPanel() { echo "Welcome to the Admin Panel!"; } } $admin = new AdminUser("Bob", "[email protected]"); $admin->greet(); // Inherited from User $admin->accessAdminPanel();
- Polymorphism: The ability of objects of different classes to respond to the same message (method call) in their own unique way. PHP
class Cat { public function makeSound() { echo "Meow!"; } } class Dog { public function makeSound() { echo "Woof!"; } } function animalSound( $animal ) { $animal->makeSound(); } $cat = new Cat(); $dog = new Dog(); animalSound( $cat ); // Output: Meow! animalSound( $dog ); // Output: Woof!
- Abstraction: Simplifying complex systems by modeling classes based on their essential characteristics and behaviors, hiding unnecessary implementation details.
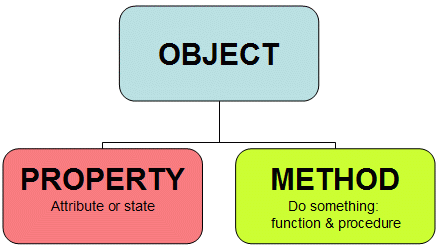
User-Focused Software and OOP
OOP excels in building user-focused software due to its inherent ability to model real-world scenarios and user interactions:
- User Profiles and Personalization: Model user accounts, preferences, and behaviors using classes. This allows for tailored content, recommendations, and user experiences.
- E-commerce Systems: Represent products, shopping carts, orders, and payment gateways as classes. OOP facilitates seamless interactions between these components, providing users with a smooth shopping experience.
- Social Networking Platforms: Model users, posts, comments, likes, and shares as objects. OOP streamlines the management of user interactions and content organization.
- Content Management Systems (CMS): Structure content, users, and permissions using classes. OOP enables flexible content creation, editing, and publishing workflows.
- Gaming Applications: Represent game entities (players, enemies, objects) as classes. OOP allows for complex interactions, game logic, and dynamic behavior.
Benefits of OOP in User-Focused Software
- Improved User Experience: Modular and well-organized code leads to more responsive, intuitive, and user-friendly applications.
- Enhanced Maintainability: Code is easier to understand, modify, and extend, ensuring that your software can adapt to evolving user needs.
- Scalability: OOP facilitates the development of scalable applications that can handle increasing user loads and feature expansions.
- Code Reusability: Classes and objects can be reused across different projects, saving development time and effort.
Advanced OOP Concepts
Let’s delve into a few more advanced OOP features you can leverage:
- Interfaces: Define contracts that classes must adhere to, promoting loose coupling and flexibility.
- Abstract Classes: Provide a base structure for related classes, enforcing certain methods while allowing for implementation variations.
- Traits: Enable code reuse by sharing methods across multiple classes without inheritance.
- Static Methods and Properties: Belong to the class itself, not to any specific object instance.
- Magic Methods: Special methods triggered automatically in response to specific events (e.g.,
__construct
,__destruct
,__toString
).
Example (Interfaces and Abstract Classes):
interface Notifiable {
public function sendNotification($message);
}
abstract class User {
// ... (properties and methods)
abstract public function getRole();
}
class RegisteredUser extends User implements Notifiable {
// ...
public function getRole() {
return 'Registered User';
}
public function sendNotification($message) {
// Implementation for sending notifications to registered users
}
}
Example (Traits):
trait Loggable {
public function logActivity($action) {
// Implementation for logging user activity
}
}
class AdminUser extends User {
use Loggable;
// ...
}
$admin = new AdminUser("Bob", "[email protected]");
$admin->logActivity('Accessed admin panel');
Example (Static Methods and Properties):
class Database {
private static $connection;
public static function getConnection() {
if (self::$connection === null) {
// Establish database connection
}
return self::$connection;
}
}
$dbConnection = Database::getConnection();
Incorporating into User-Focused Software
These advanced OOP techniques empower you to craft even more sophisticated and user-centric applications:
- User Roles and Permissions: Utilize inheritance and interfaces to model different user types (e.g., admin, moderator, regular user) with varying levels of access and capabilities.
- Notifications and Event Handling: Employ interfaces and traits to implement notification systems or event-driven architectures, providing users with real-time updates and personalized interactions.
- Data Access and Persistence: Leverage static methods and abstract classes to manage database connections and interactions, ensuring data integrity and efficient data retrieval for user-related operations.
Conclusion
Object-Oriented Programming in PHP is a cornerstone of modern software development, particularly when creating user-focused applications. By embracing OOP principles, you can model real-world entities, manage complex interactions, and provide users with seamless and engaging experiences.
As you embark on your PHP development journey, remember to leverage the power of classes, objects, and OOP concepts to build software that truly resonates with your users.